Overview
Purpose: adds left and right arrow buttons to a carousel-like component like Carousel.
This mixin does most of its work at the beginning of the Elix render pipeline:
events ➞ methods → setState → render DOM → post-render
Expects the component to provide:
internal.canGoLeft
andinternal.canGoRight
properties, typically via DirectionCursorMixin. These properties determine when the arrow buttons are enabled or disabled. If the component does not define these properties, the arrow buttons are always enabled.internal.goLeft
andinternal.goRight
methods, typically via DirectionCursorMixin.internal.template
property for ShadowTemplateMixin. The property getter must invoke the mixin'swrapWithArrowDirection
(below).
Provides the component with:
wrap
method that destructively wraps a template with the elements for left and right arrow buttons.
Usage
import { ArrowDirectionMixin, ReactiveElement, internal, template } from "elix";
class MyElement extends ArrowDirectionMixin(ReactiveElement) {
get [internal.template]() {
const t = template.html`
<div id="container">
<slot></slot>
</div>
`;
// Wrap the indicated container with arrows.
const container = t.content.querySelector("#container");
this[ArrowDirectionMixin.wrap](container);
return t;
}
}
ArrowDirectionMixin
is a mixin that contributes to a component's template, as shown above.
As currently implemented, ArrowDirectionMixin
only displays the arrow buttons if mouse movement is detected. This behavior is intended to avoid showing arrows when the user is viewing a carousel-like component on a mobile device with no mouse. In such cases, it is expected that the component will provided touch gestures (e.g., with TouchSwipeMixin), so the arrows are unnecessary and may prove distracting or reduce the screen real estate available for touch gestures.
Customizing
In addition to custom styling via template patching or the internal.render method, ArrowDirectionMixin
supports the following customizations:
- arrowButton property: specifies the element role used for the left and right arrow buttons.
arrowButtonLeft
slot: sets the content of the left arrow button.arrowButtonRight
slot: sets the content of the right arrow button.
Example
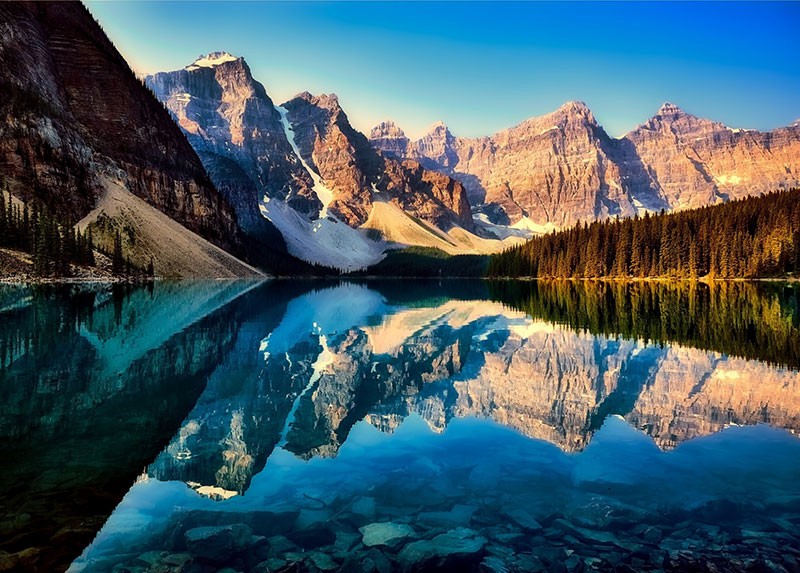
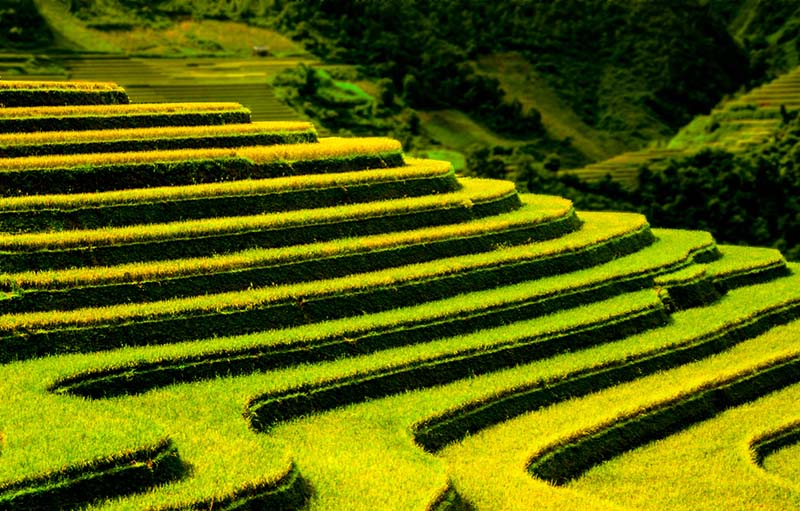
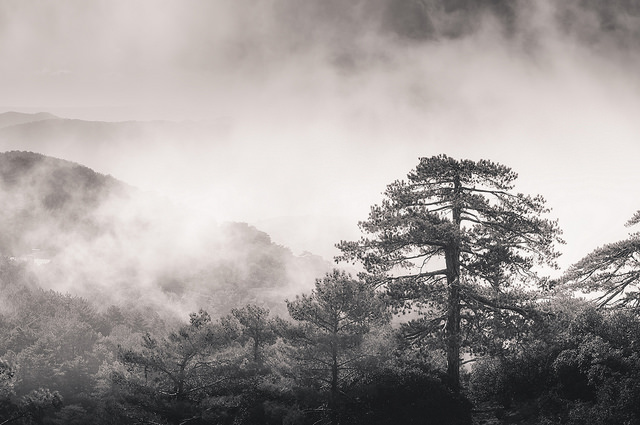
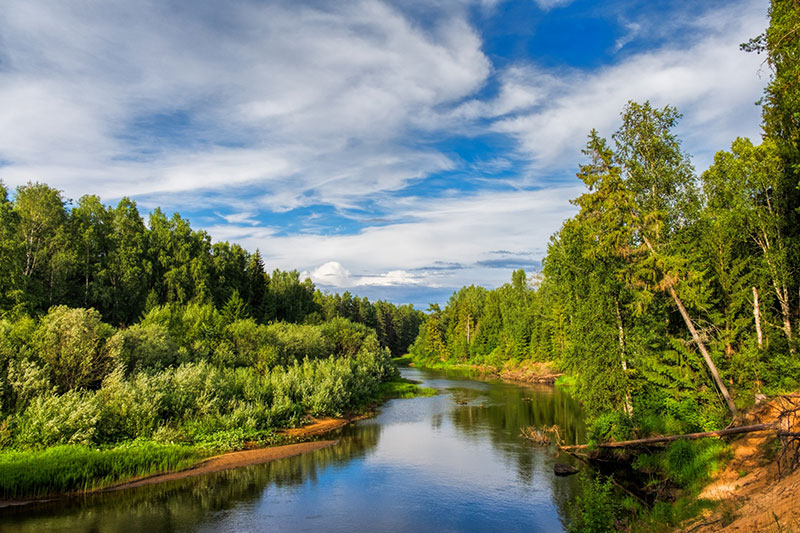
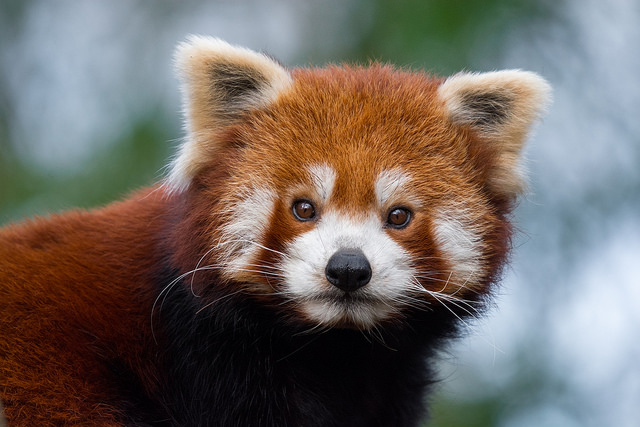
API
The element defines the following shadow parts:
-
arrow-button
: both of the arrow buttons, default type is Button -
arrow-button-next
: the arrow button that navigates to the next item -
arrow-button-previous
: the arrow button that navigates to the previous item
Used by classes CalendarMonthNavigator, Carousel, CarouselSlideshow, CarouselWithThumbnails, PlainCalendarMonthNavigator, PlainCarousel, PlainCarouselSlideshow, and PlainCarouselWithThumbnails.
arrow Button Overlap property
True if the arrow buttons should overlap the component contents; false if they should appear to the side of the contents.
Type: boolean
Default: true
arrow Button Part Type property
The class or tag used to create the arrow-button
parts – the
previous/next arrow buttons.
Type: (component class constructor)|HTMLTemplateElement|string
wrap(target) method
Destructively wrap a node with elements to show arrow buttons.
Parameters:
- target:
Element
– the node that should be wrapped by buttons