Overview
Purpose: adds buttons for managing playback of a slideshow, audio playlist, etc.
This mixin does most of its work at the beginning of the Elix render pipeline, although it also participates indirectly in rendering by adding elements to a component's template.
events ➞ methods → setState → render DOM → post-render
Expects the component to provide:
playing
property andplay
andpause
methods, typically via TimerCursorMixin.
Provides the component with:
wrap
method that wraps a string template with the elements for the play controls.
Usage
import { PlayControlsMixin, internal, template } from "elix";
class MyElement extends PlayControlsMixin(HTMLElement) {
get [internal.template]() {
const result = template.html`
<div id="container">
<slot></slot>
</div>
`;
// Wrap the indicated container with play controls.
const container = result.content.querySelector("#container");
this[PlayControlsMixin.wrap](container);
return result;
}
}
Example
SlideshowWithPlayControls uses this mixin for its play controls:
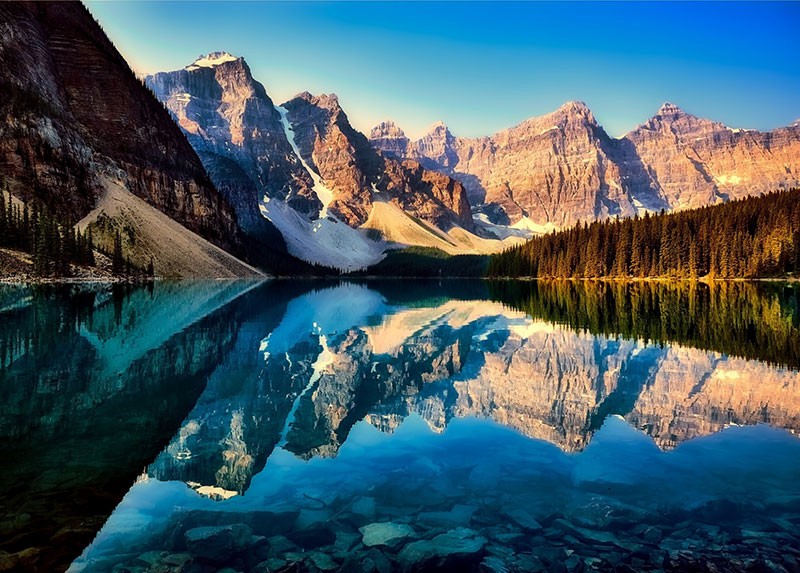
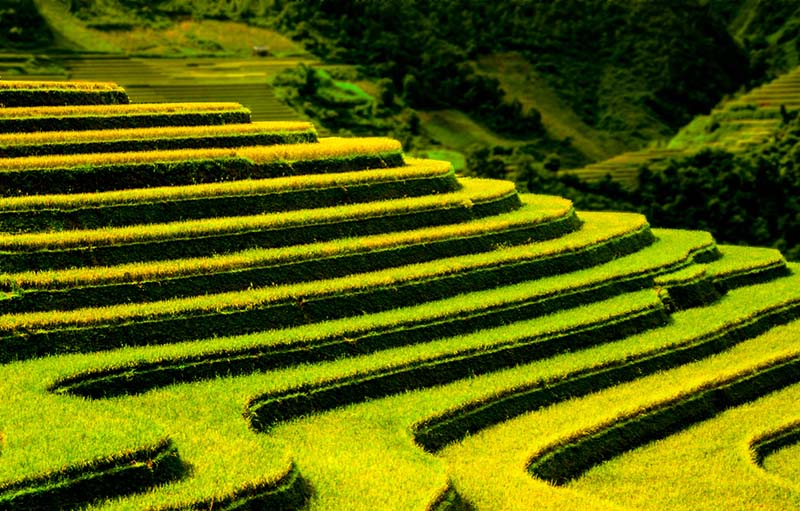
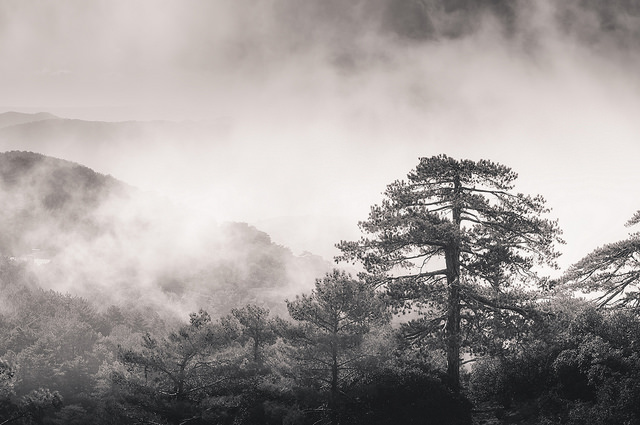
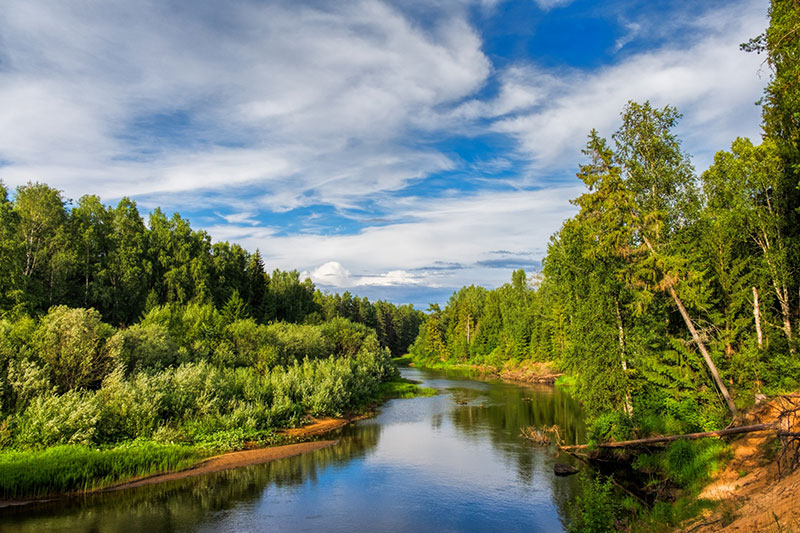
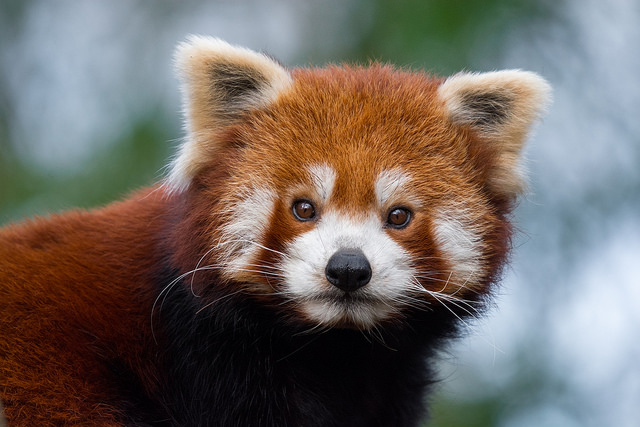
API
The element defines the following shadow parts:
-
control-button
: any of the buttons that control playback, default type is Button -
next-button
: the button that navigates to the next item -
play-button
: the button that starts or pauses playback -
previous-button
: the button that navigates to the previous item
Used by classes PlainSlideshowWithPlayControls and SlideshowWithPlayControls.
control Button Part Type property
The class or tag used to create the control-button
parts –
the play control buttons.
Type: (component class constructor)|HTMLTemplateElement|string
Default: Button
wrap(target) method
Destructively wrap a node with elements for play controls.
Parameters:
- target:
Element
– the element that should be wrapped by play controls