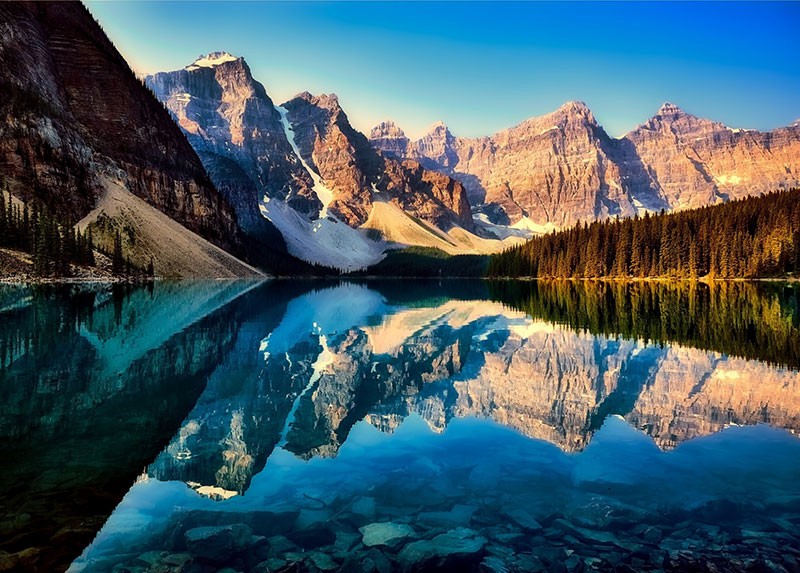
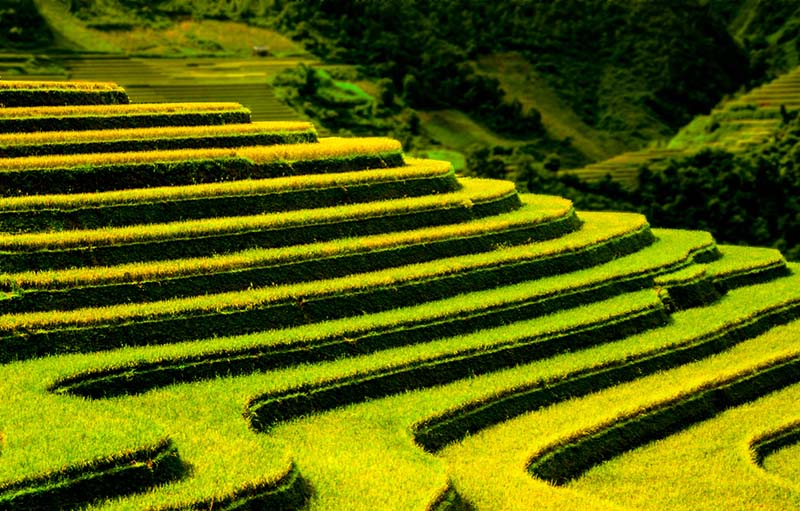
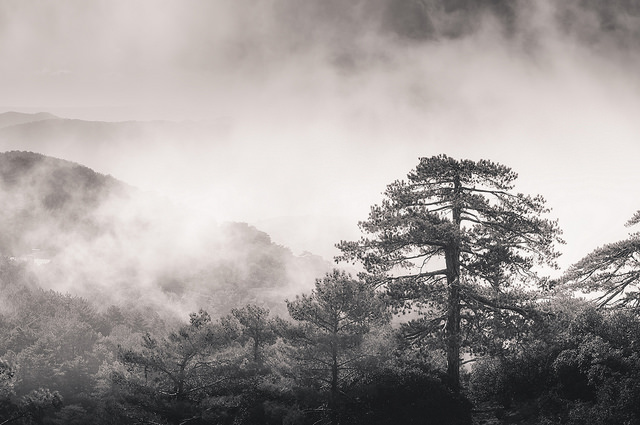
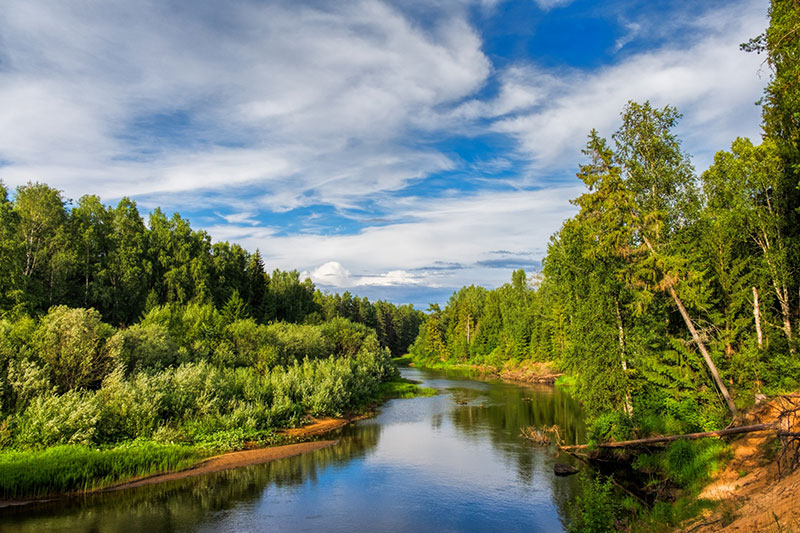
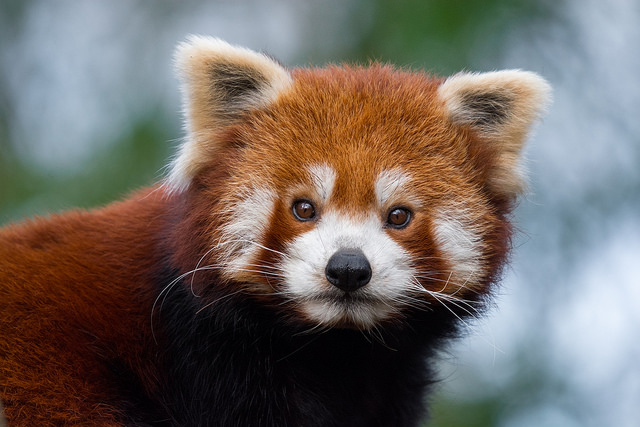
Overview
Purpose: Lets a component automatically or explicitly configure itself for dark backgrounds.
This mixin works at the beginning of the Elix render pipeline:
events ➞ setState → render DOM → post-render
Expects the component to provide:
setState
method compatible withReactiveMixin
's setState.
Provides the component with:
dark
property that is true if the component should show its dark mode, false if not.detectDarkMode
property. If "auto" (the default), the component should setdark
automatically on a dark background.- Tracking of the current state of the prefers-color-scheme CSS media feature.
Usage
import ReactiveElement from "elix/src/core/ReactiveElement.js";
import DarkModeMixin from "elix/src/base/DarkModeMixin.js";
class MyElement extends DarkModeMixin(ReactiveElement) {}
By default, a component using DarkModeMixin
will set its dark
property if the component is added to a container with a dark background. The mixin considers any color with a HSL luminance value less than 50% to be dark, otherwise the color is considered to be light.
You can make a component instance responsive to the standard prefers-color-scheme
CSS media feature. Define a CSS rule of the component instance or a container element (like body) that sets the background color based on prefers-color-scheme
:
body {
background: white;
}
@media (prefers-color-scheme: dark) {
body {
background: black;
}
}
The component will detect the initial background color and set dark
if its on a dark background. If the user changes their OS color scheme preference, the component will detect that and recalculate dark
from the new background color.
DarkModeMixin
does not respond to changes in background color that happen for other reasons.
Example
These two PlainCarousel components are generally identical, except that the second has been given a dark background color, and will default to dark mode:
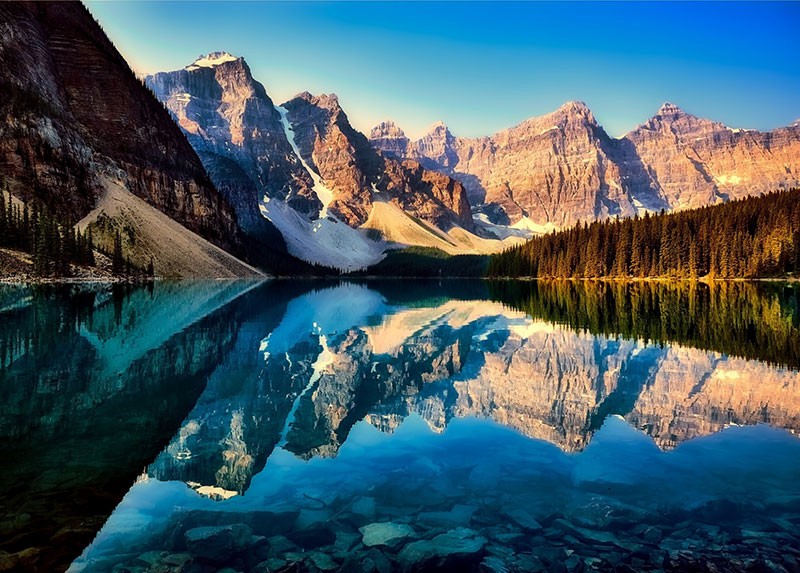
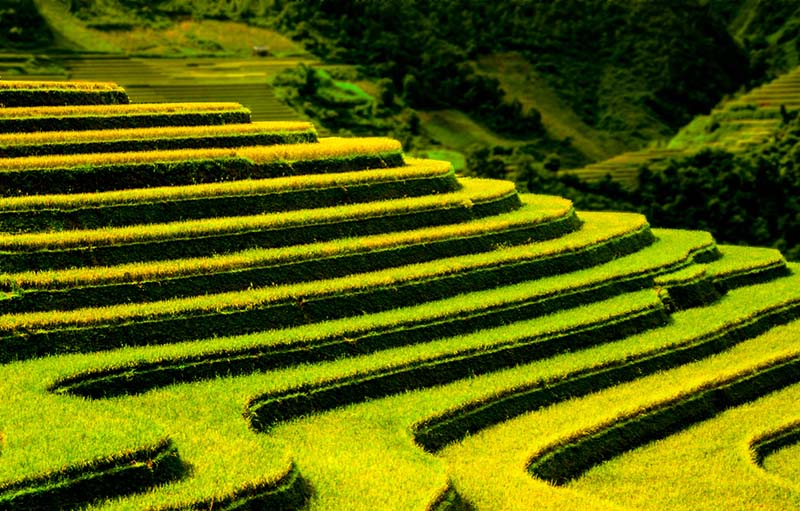
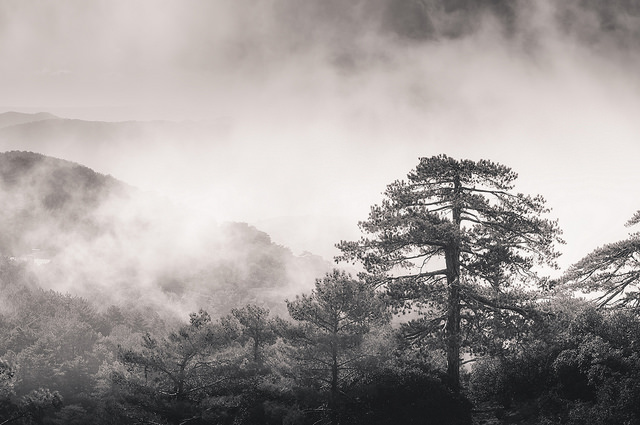
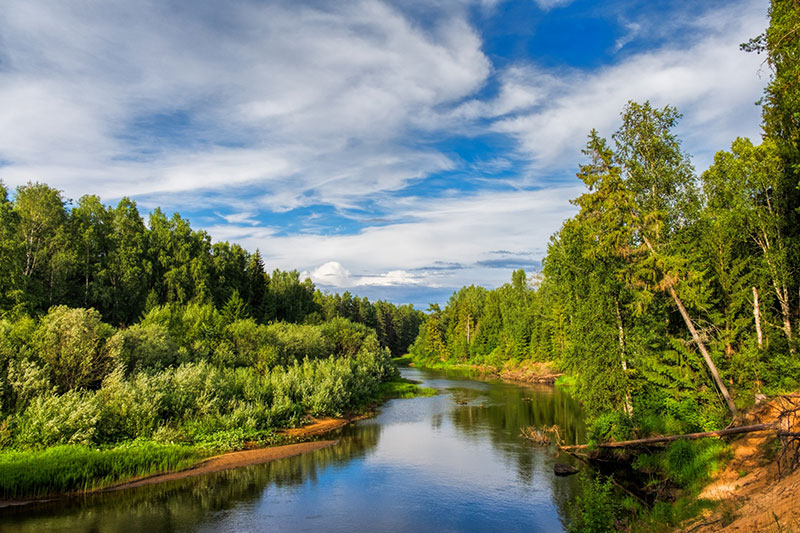
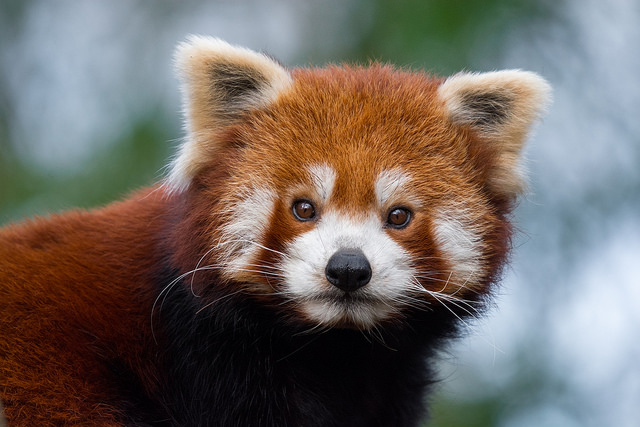
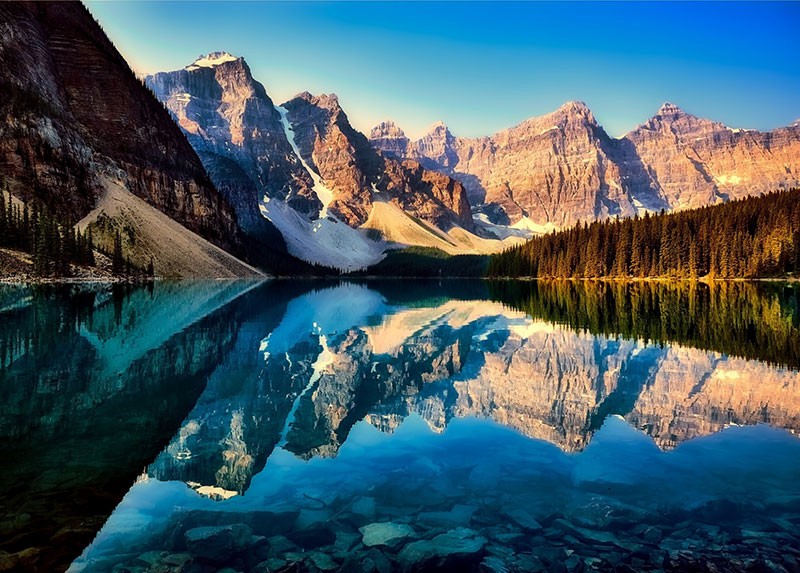
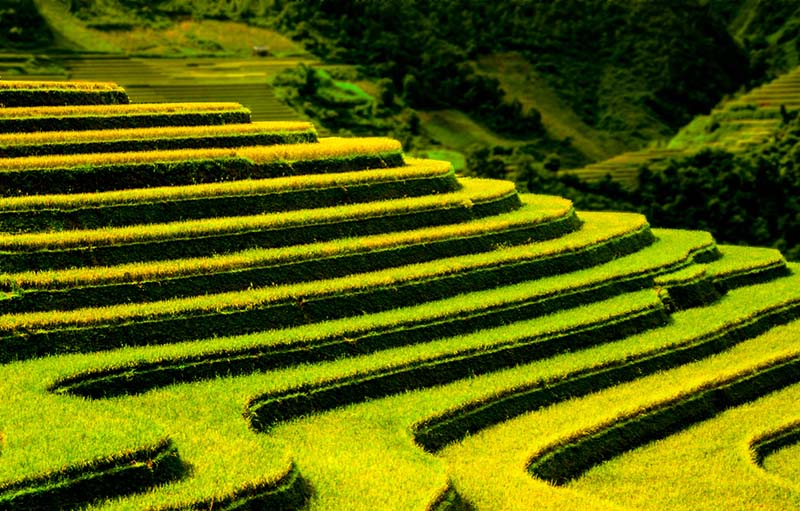
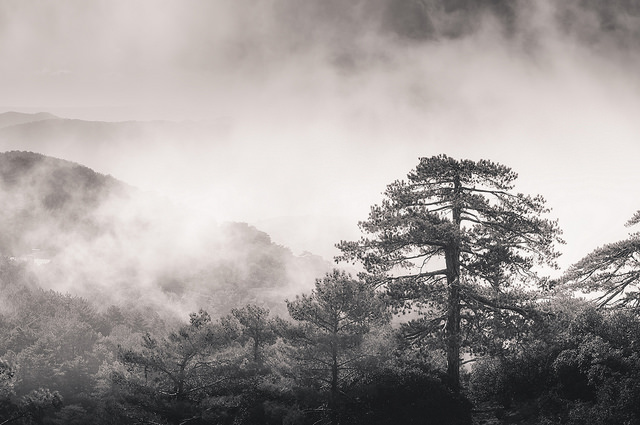
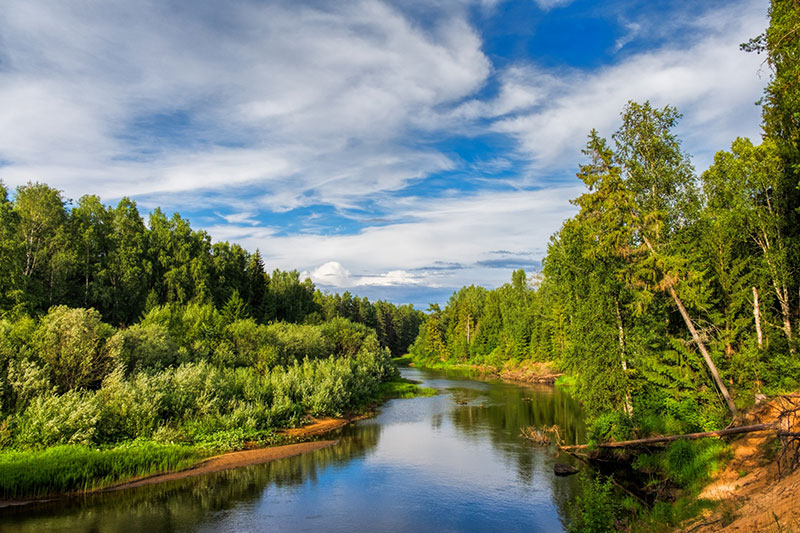
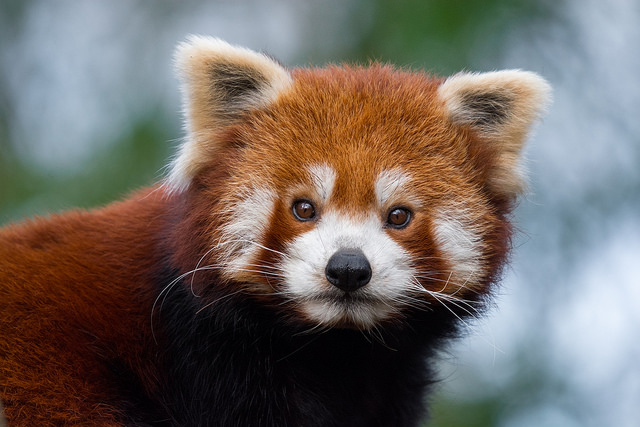
Since the second carousel defaults to dark mode, its interior PlainArrowDirectionButton and PlainPageDot elements will be set for dark mode. This entails using light foreground colors in all element states, including disabled, hover, and selected states.
API
Used by classes PlainArrowDirectionButton, PlainCalendarMonthNavigator, PlainCarousel, PlainCarouselSlideshow, PlainCarouselWithThumbnails, and PlainPageDot.
dark property
True if the component should configure itself for display on a dark background; false if the component should assume a light background.
The default value of this property is inferred when the component is
initially added to the page. The component will look up its hierarchy for
an ancestor that has an explicit background color. If the color's
lightness value in the HSL cylindrical-coordinate system is below 50%,
the background is assumed to be dark and dark
will default to true.
If the color is lighter than that, or no explicit background color can be
found, the default value of dark
will be false.
Type: boolean
detect Dark Mode property
Determines whether the component should automatially try to detect whether it should apply dark mode.
Type: 'auto'|'off'
Default: 'auto'